Introduction
Welcome to "Getting Started with Python for AI: A Comprehensive Beginner’s Guide." This tutorial is your gateway to the world of Python programming and artificial intelligence (AI). Whether you’re new to coding or eager to explore AI, this guide will provide you with the foundational knowledge and practical skills you need to get started. We’ll cover Python basics, introduce essential AI libraries, and walk you through your first AI project. By the end, you’ll have a solid understanding of Python for AI and be ready to take on more advanced topics.
Table of Contents
- Why Python for AI?
- Setting Up Your Python Environment
- Understanding Python Basics
- Introduction to Libraries for AI
- Your First AI Project: Sentiment Analysis
- Next Steps: Exploring Advanced AI Concepts
- Interactive Learning Resources
1. Why Python for AI?
Python is the most popular language for AI development, favored by both beginners and professionals. Its clean syntax, extensive libraries, and strong community support make it an excellent choice for anyone looking to dive into AI. Python allows you to transition smoothly from writing basic scripts to implementing complex algorithms, all within the same ecosystem.
Related Reading: Why Python is Perfect for AI Development
2. Setting Up Your Python Environment
To begin your journey into Python for AI, you need to set up a development environment:
- Install Python:
Download the latest version of Python from python.org. Ensure Python is added to your PATH during installation.
Install Necessary Packages:
pip install numpy pandas scikit-learn matplotlib
Create a Virtual Environment:
python -m venv ai_env
source ai_env/bin/activate # On Windows use `ai_env\Scripts\activate`
3. Understanding Python Basics
Understanding Python basics is crucial as you move into AI. Here’s a quick overview:
Functions and Modules:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Control Structures:
if age > 18:
print("Adult")
else:
print("Minor")
Variables and Data Types:
age = 25
name = "Alice"
is_student = True
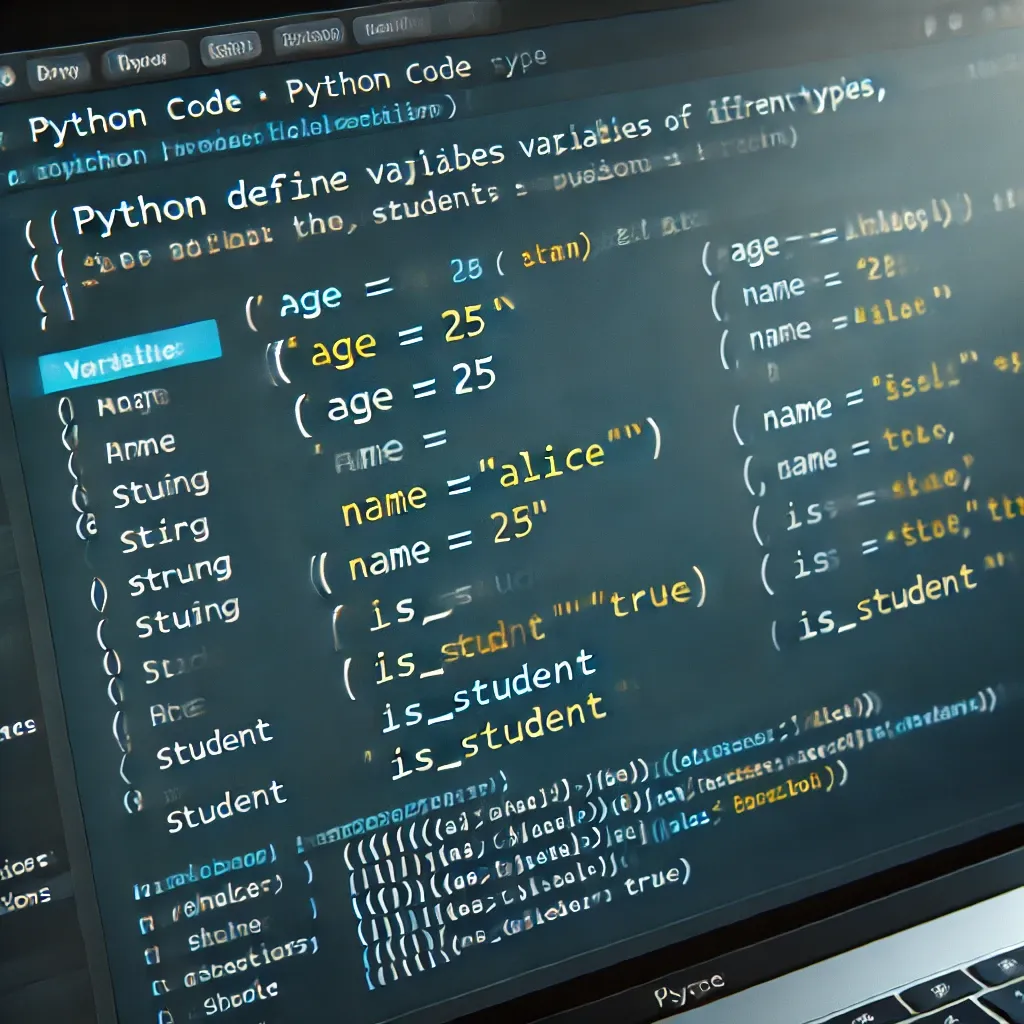
External Resource: Python Basics Tutorial
4. Introduction to Libraries for AI
Python's power in AI comes from its rich set of libraries. Let’s explore a few essential ones:
Scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
Further Reading: Scikit-learn Documentation
Matplotlib:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [10, 20, 25, 30])
plt.title("Simple Plot")
plt.show()
Interactive Plotting: Try out your own plots on Google Colab.
NumPy and Pandas:
import numpy as np
import pandas as pd
data = np.array([1, 2, 3, 4, 5])
df = pd.DataFrame(data, columns=["Numbers"])
print(df)
Video Tutorial: Introduction to NumPy and Pandas
5. Your First AI Project: Sentiment Analysis
Sentiment Analysis is a great beginner project in AI. Here’s how to get started:
- What is Sentiment Analysis?
Sentiment Analysis uses Natural Language Processing (NLP) to analyze and classify text as positive, negative, or neutral. It's widely used in social media monitoring and customer feedback analysis. - Interpreting the Results:
The output will show whether the sentiment is positive or negative, along with a confidence score. You can experiment with different text inputs to see how the model performs.
Writing and Running Your Python Code:
Here’s how to implement a simple sentiment analysis:
from transformers import pipeline
sentiment_pipeline = pipeline("sentiment-analysis")
data = ["I love Python!", "I hate bugs."]
sentiments = sentiment_pipeline(data)
for sentiment in sentiments:
print(f"Text: {data[sentiments.index(sentiment)]}, Sentiment: {sentiment['label']}, Score: {sentiment['score']:.2f}")
Interactive Notebook: Run the code yourself on this Google Colab Notebook.
Setting Up Your Project:
Start by installing the necessary library:
pip install transformers
Link to Advanced Projects: Building Advanced Sentiment Analysis Models
6. Next Steps: Exploring Advanced AI Concepts
Now that you’ve built your first AI project, here’s what you can explore next:
- Deep Learning with TensorFlow or PyTorch: These frameworks allow you to build complex neural networks.
- External Resource: TensorFlow Beginner’s Guide.
- Natural Language Processing (NLP): Dive deeper into NLP with libraries like spaCy and NLTK.
- Interactive Course: NLP with Python
- Computer Vision: Explore image processing and recognition with OpenCV.
- Interactive Guide: OpenCV for Beginners
Interactive Element: Take a quiz to test your understanding of Python basics and AI concepts. Start Quiz
7. Interactive Learning Resources
To continue your learning journey, explore these interactive resources:
- Code Challenges: Practice Python coding with real-time feedback on HackerRank.
- AI Simulations: Experiment with AI models using Google AI Experiments.
- Discussion Forums: Join discussions with other learners on Stack Overflow and Reddit’s AI Community.
Internal Links for Further Learning:
You’ve taken your first steps into the exciting world of Python and AI. This guide has equipped you with the knowledge to start building AI projects on your own. Remember, the key to mastering AI is continuous learning and experimentation.
Don’t forget to subscribe to our newsletter for more tutorials, tips, and resources on Python and AI.